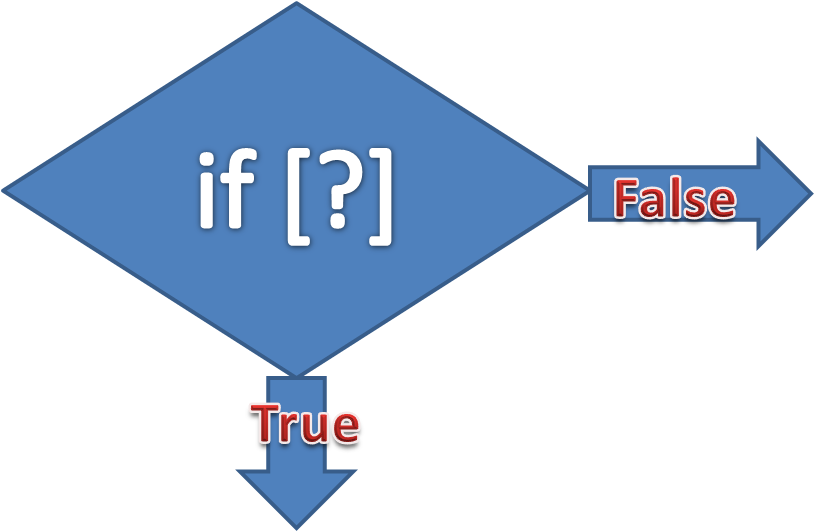
1. Bash if Statement
Single if statements are useful where we have a single program for execution.
if [ condition ] then < code block > fi
For example – If we need to check if input value is equal to 10 or not. If value is equal to 10, then it will print “Value of i is 10″, but if not nothing will be printed.
#!/bin/bash read -p "Enter value of i :" i if [ $i -eq 10 ] then echo "Value of i is 10" fi
2. Bash if else Statement
if else statements are useful where we have a two programs for execution, and need to execute only one based on results of if condition.
if [ condition ] then < code block > else < code block > fi
For example – If input taken value is 10 then it will print “Value of i is 10″, if not program will execute else block statement and print “Value of i is not equal to 10″.
#!/bin/bash read -p "Enter value of i :" i if [ $i -eq 10 ] then echo "Value of i is 10" else echo "Value of i is not equal to 10" fi
3. Bash if elif Statement
if elif and else statements are useful where we have more than two programs for execution, and need to execute only one based on results of if and elif condition.
if [ condition ] then < code block > elif [ condition ] then < code block > else < code block > fi
For example – Below example will check input value if equal to 5, if its true then program will print “Value of i is 5″ otherwise program will go to elif statement where one more conditional will be checked and based of these results elif or elase block code will be executed.
#!/bin/bash read -p "Enter value of i :" i if [ $i -eq 5 ] then echo "Value of i is 5" elif [ $i -eq 10 ] then echo "Value of i is 10" else echo "Value of i is not equal to 5 or 10" fi
4. Bash elif Ladder Statements
This is something similar to above one where we are adding multiple elif statements together. elif (else if) ladder are useful where we have multiple programs for execution and need to execute only one based on results of if and elif condition.
if [ condition ] then < code block > elif [ condition ] then < code block > elif [ condition ] then < code block > elif [ condition ] then < code block > else < code block > fi
For example –
#!/bin/bash read -p "Enter value of i :" i if [ $i -eq 5 ] then echo "Value of i is 5" elif [ $i -eq 10 ] then echo "Value of i is 10" elif [ $i -eq 20 ] then echo "Value of i is 20" elif [ $i -eq 30 ] then echo "Value of i is 30" else echo "Value of i is not equal to 5,10,20 or 30" fi
5. Bash nested if Statements
Nested if are useful in the situation where one condition will be checked based on results of outer condition.
if [ condition ] then if [ condition ] then < code block > else < code block > fi else if [ condition ] then < code block > fi fi
For example below small shell program is for finding the greatest value between 3 values taken input by user. This program will work with numeric values only. If two values are similar it will print only one value.
#!/bin/bash read -p "Enter value of i :" i read -p "Enter value of j :" j read -p "Enter value of k :" k if [ $i -gt $j ] then if [ $i -gt $k ] then echo "i is greatest" else echo "k is greatest" fi else if [ $j -gt $k ] then echo "j is greatest" else echo "k is greatest" fi fi
Leave a Reply
You must be logged in to post a comment.